Simple Logger
SimpleLogger is a C# application logger that can easily be plugged into your application to provide flexible logging. It uses various design patterns to build this component from the Strategy Pattern to the Abstract Factory Pattern.Since this is a .Net component, it can be used in an ASP.NET web application, a WinForms application, and ASP.NET MVC application, etc.
Here's a look at the Visual Studio solution that shows the SimpleLogger project and a sample ASP.NET web application that uses it.
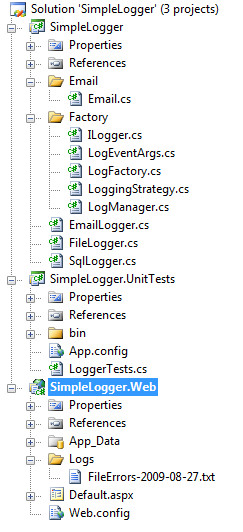
This is an example of how it would be used in a click event of an ASP.NET web application.
01.
protected
void
btnCreateError_Click(
object
sender, EventArgs e)
02.
{
03.
try
04.
{
05.
throw
new
Exception(
"This is an exception Thrown by King Wilder."
);
06.
}
07.
catch
(Exception ex)
08.
{
09.
// This single line will log to the destination of your choice.
10.
LogManager.WriteMessage(
new
LogEventArgs(
"SimpleLogger.Web._Default.btnCreateError_Click"
, ex.Message, ex));
11.
12.
lblMessage.Text = ex.Message;
13.
GridView1.DataBind();
14.
}
15.
}
Line 10 shows the single line of code for the logger. This takes a LogEventArgs object as an argument to the WriteMessage static method. The WriteMessage method has a few overloads that take various parameters:
- Method name - this can be anything but it should be the name of the method
- Error message - this is the error message from the Exception
- Exception - this is the Exception itself
This is an example of how you can configure the LogTypes in the appSettings section.
1.
<
appSettings
>
2.
<!-- Available Options are: FileError, FileTrace, Sql, Event -->
3.
<
add
key
=
"LogTypes"
value
=
"FileError,Sql"
/>
4.
<
add
key
=
"connName"
value
=
"ErrorLogConnection"
/>
5.
</
appSettings
>
Line 2 shows the various LogTypes that are available, and you just enter the log types that you want to set. For the SQL log type, you will of course need to create the data store and have the connection to the database set in your web.config.
This is the code for the LogManager class that contains the static WriteMessage method.
01.
using
System;
02.
using
System.Collections.Generic;
03.
using
System.Configuration;
04.
05.
namespace
SimpleLogger
06.
{
07.
/// <summary>
08.
/// A static class to implement log management from SimpleLogger.
09.
/// </summary>
10.
public
class
LogManager
11.
{
12.
/// <summary>
13.
/// Log a message to all the listed ILogger subclasses.
14.
/// </summary>
15.
/// <param name="args"></param>
16.
public
static
void
WriteMessage(LogEventArgs args)
17.
{
18.
string
[] types =
19.
ConfigurationManager.AppSettings.Get(
"LogTypes"
).Split(
','
);
20.
21.
List<LoggingStrategy> strategyList =
new
List<LoggingStrategy>();
22.
foreach
(
string
type
in
types)
23.
{
24.
LoggingStrategy logType =
25.
(LoggingStrategy)Enum.Parse(
typeof
(LoggingStrategy), type,
true
);
26.
strategyList.Add(logType);
27.
}
28.
29.
// Implement the factory pattern to return the proper Logger subclasses.
30.
LogFactory factory =
new
LogFactory();
31.
List<ILogger> loggers = factory.CreateLoggers(strategyList);
32.
33.
int
i = 0;
34.
// Iterate through the list of loggers and log the messages.
35.
foreach
(ILogger logger
in
loggers)
36.
{
37.
ILogger log = logger;
38.
39.
args.LogType = types[i];
40.
41.
// Log the message.
42.
log.Log(args);
43.
i++;
44.
}
45.
}
46.
}
47.
}
Line 30 shows the LogFactory class that reads the LogTypes of the appSettings section of the web.config file and determines all the loggers to create.
The nice thing about this component is that you can create more than one logger at a time.